What is an Arrow Function in JavaScript– An arrow function in JavaScript is a modern and concise way to write function expressions, introduced in ES6 (ECMAScript 2015). It provides a more compact syntax than traditional functions and offers unique behavior about the this
context. Arrow functions are often favored for their simplicity and readability, especially when dealing with one-liner functions or callbacks.
Table of Contents
1. Arrow Function Syntax
The syntax for an arrow function is quite simple. You use the arrow (=>
) to separate the parameters from the function body. The basic structure looks like this:

If you have a single expression in the function, the curly braces and the return
keyword can be omitted. Here is an example of an arrow function that adds two numbers:

2. Key Characteristics: What is an Arrow Function in JavaScript?
Arrow functions differ from traditional JavaScript functions in several important ways:
Concise Syntax
Arrow functions offer a more compact way of defining functions. If the body contains just a single expression, the curly braces and return
a keyword can be left out, making it ideal for short functions.
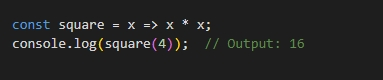
Implicit Return
When you write an arrow function without curly braces, the value of the expression is automatically returned. There is no need to explicitly use the return
keyword.
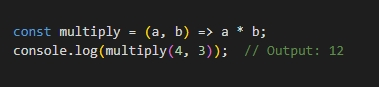
No Own this
Context
One of the most important differences is that arrow functions do not have their own this
context. Instead, they inherit this
from their surrounding lexical context. This is beneficial in situations where traditional functions might behave unexpectedly due to how this
is determined by the call site.
Example of an issue with traditional functions:
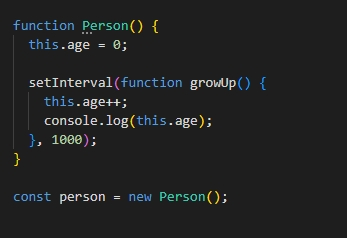
In this case, this
in the growUp
function does not refer to the Person
object, but to the global object. However, if you use an arrow function, this
will refer to the surrounding lexical context:
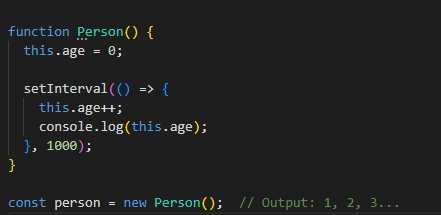
Cannot be used as Constructors
Arrow functions cannot be used as constructors and will throw an error if you attempt to use them with the new
keyword. They are not designed to be used for object instantiation.
No arguments
Object
Unlike traditional functions, arrow functions do not have their arguments
own object. However, you can achieve similar behavior by using the rest parameters (...args
).
3. Benefits of Using Arrow Functions
- Conciseness: The shorter syntax is more readable, especially for simple functions or callbacks.
- Lexical
this
: Arrow functions inherit the valuethis
from their surrounding context, making them a great fit for functions that are nested or used as callbacks. - Implicit return: One-liners become very clean when the return of a keyword is not necessary.
4. Limitations of Arrow Functions
- No binding of
this
: Arrow functions cannot be used in cases where the function’s context (this
) needs to dynamically change. - No
new
keyword: They cannot be used as constructors, which limits their use when creating new instances of objects. - No
arguments
object: Arrow functions do not have anarguments
object, which could be a limitation when dealing with variable numbers of arguments without rest parameters.
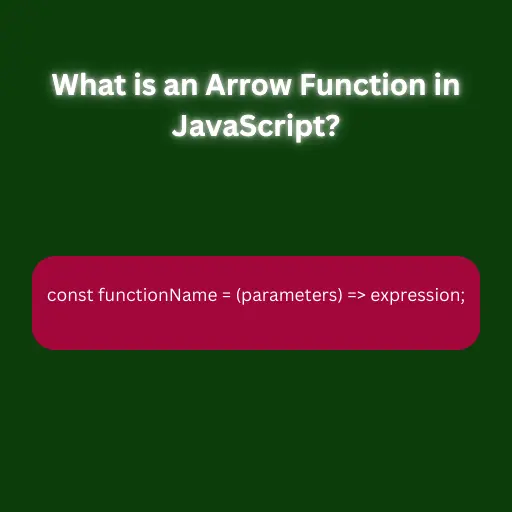
Comparison of Arrow Functions vs. Traditional Functions
Feature | Arrow Function | Traditional Function |
---|---|---|
Syntax | Concise, uses => | Longer, uses function keyword |
this context | Inherits from the surrounding context | Determined by how the function is called |
arguments object | Not available | Available |
Use with new | Not allowed | Allowed |
Return syntax | Implicit return for single expressions | Requires return for any value |
1. What is an Arrow Function in JavaScript?
An arrow function is a more concise syntax for writing functions in JavaScript. It was introduced in ES6 and has several unique characteristics, such as not having its own this
context.
2. How do arrow functions handle this
differently from regular functions?
Arrow functions do not have their own this
context. They inherit this
from the surrounding lexical scope, which makes them useful in callbacks and event handlers.
3. Can arrow functions be used as constructors?
No, arrow functions cannot be used as constructors, and attempting to use the new
keyword with them will result in an error.
4. What is an implicit return in an arrow function?
If an arrow function has only a single expression and no curly braces, the expression is returned automatically without needing the return
keyword.