Difference Between let, var, and const in JavaScript: A Complete Guide- In JavaScript, var
, let
, and const
are the three primary ways to declare variables. Each behaves differently in terms of scope, hoisting, and re-declaration rules. Understanding these differences is essential for writing clean, bug-free code.
This guide breaks down the key, Differences between let, var, and const in JavaScript with examples, best practices, and a comparison table to help you understand when to use each.
Table of Contents
1. var
in JavaScript: The Traditional Way
The var keyword has been around since the early days of JavaScript, and while it still works, it has some quirks that can lead to unexpected behavior.
Key Characteristics of var
: Difference Between let, var, and const
Scope: var
is function-scoped, meaning it’s confined to the function where it’s declared. If declared outside any function, it becomes globally scoped.
Hoisting: Variables declared var
are hoisted, meaning they are moved to the top of their scope at runtime. However, they are initialized, so accessing them before declaration will return undefined
.
Re-declaration: You can redeclare a var
variable without an error.
Block Scoping: var
ignores block scoping. If declared inside{}, the variable is accessible outside the block.
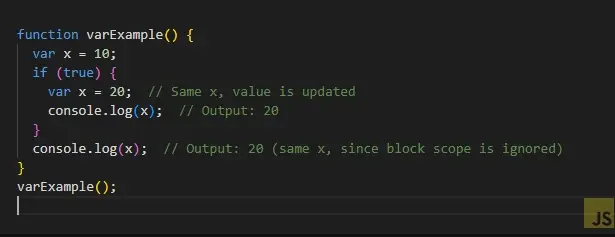
2. let
in JavaScript: Block-Scoped and Modern
Introduced in ES6 (ECMAScript 2015), let
improves variable scoping and reduces the issues seen with var
. It’s recommended to use let
over var
in most cases.
Key Characteristics of let
:
- Scope:
let
is block-scoped, meaning it’s limited to the block{}
where it is declared. It is not accessible outside of that block. - Hoisting: Like
var
,let
is hoisted. However, it is not initialized until its declaration, so accessing it before the declaration results in aReferenceError
. - Re-declaration: You cannot redeclare a
let
variable within the same scope, which helps prevent accidental reassignments. - Block Scoping:
let
respects block scope, meaning variables inside{}
are confined to that block.
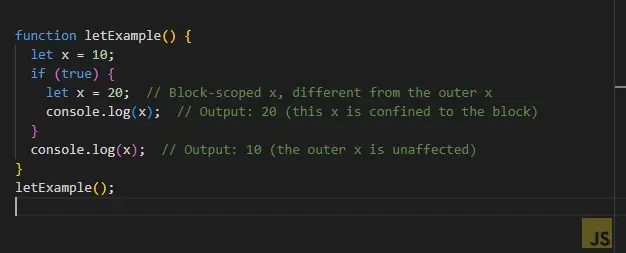
3. const
in JavaScript: For Constants
Also introduced in ES6, const
is used to declare constants—variables whose values cannot be reassigned after they are initialized. However, note that const
does not make the contents of arrays or objects immutable; it only ensures that the variable itself cannot be reassigned.
Key Characteristics of const
:
- Scope: Like
let
,const
is block-scoped. - Hoisting:
const
is hoisted but not initialized until the line of declaration, meaning accessing it before declaration results in aReferenceError
. - Re-declaration: You cannot redeclare a
const
variable in the same scope. - Immutability: You cannot change the value of a
const
variable after it is assigned. However, objects and arrays declaredconst
are mutable—you can change their contents, but you cannot reassign the variable to something else.
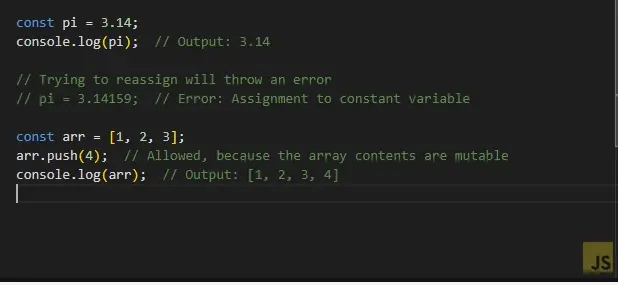
Basic Difference Between let, var, and const
Feature | var | let | const |
---|---|---|---|
Scope | Function-scoped | Block-scoped | Block-scoped |
Hoisting | Hoisted, initialized as undefined | Hoisted but not initialized | Hoisted but not initialized |
Re-declaration | Allowed in the same scope | Not allowed in the same scope | Not allowed in the same scope |
Re-assignment | Allowed | Allowed | Not allowed |
Immutability | No | No | Yes (for primitive values only) |
Q1: What is the main Difference Between let, var, and const in JavaScript?
The primary difference is in scoping. var is function-scoped, while let
is block-scoped. This means that let
variables are confined to the block {}
in which they are declared, whereas var
variables are not.
Q2: Can I use const
it for arrays and objects?
Yes, you can use const
for arrays and objects. However, while the reference to the object or array is constant (cannot be reassigned), the contents of the object or array can still be changed.
Q3: What happens if I declare a variable with var and redeclare it with let
?
If you declare a variable with var
in the same scope and try to redeclare it with let
, you will get a SyntaxError
. The reverse (declaring with let
and redeclaring with var
) will also throw an error.