How to Generate Login Form in HTML Code: Creating a login form is an essential step in web development, especially when building user authentication features for websites or applications. A well-designed login form provides users with a seamless and secure way to access their accounts. This article will guide you through generating a login form using HTML and CSS while explaining the code step by step.
Introduction to Login Forms
A login form is a gateway that allows users to access restricted areas of your website. It’s one of the most commonly used features in modern web design. The login form must be functional and visually appealing to ensure a smooth user experience.
Why Use a Login Form?
- User Authentication: Restricts access to authorized users.
- Data Security: Protects sensitive information.
- Personalized Experience: Offers tailored content based on user data.
HTML Structure of a Login Form
The foundation of any web element starts with its HTML structure. Below is the basic HTML code for a login form.
<body>
<div class="login-container">
<h2>Login</h2>
<form>
<label for="username">Username</label>
<input type="text" id="username" name="username" required>
<label for="password">Password</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
</form>
</div>
</body>
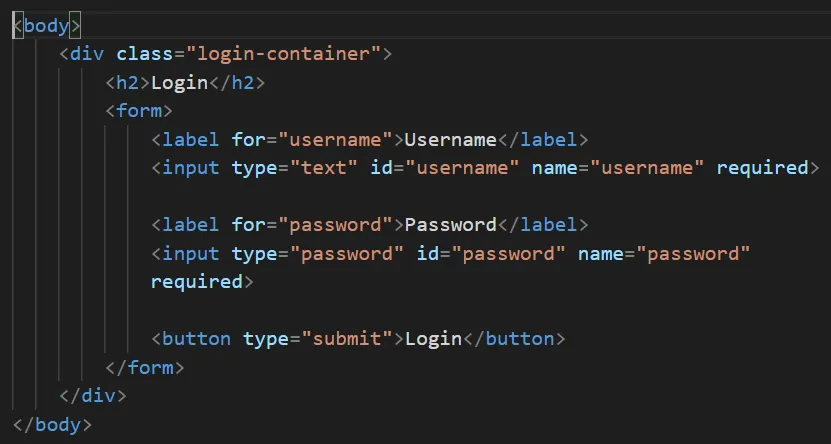
Key Features
<form>:
Acts as the container for the input fields.<label>:
Provides context for input fields.<input>:
Used for text and password inputs.<button>:
Triggers form submission.
CSS Styling for How to Generate Login Form in HTML Code
CSS transforms the plain HTML structure into an eye-catching design. Below is the CSS used to style the login form.
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background: #f4f4f9;
}
.login-container {
padding: 30px 40px;
background: #fff;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
text-align: left;
width: 320px;
}
.login-container h2 {
text-align: center;
margin-bottom: 20px;
}
label {
font-size: 14px;
margin-bottom: 5px;
color: #333;
}
input {
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 14px;
}
button {
width: 100%;
padding: 10px;
background: #007BFF;
border: none;
color: white;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background: #0056b3;
}
img
Key Styling Features
- Background and Padding: Makes the form visually distinct.
- Box Shadow: Adds a subtle 3D effect.
- Hover Effects: Enhances usability by changing button color on hover.
Enhancing Usability with Hover Effects
Hover effects make the form interactive and user-friendly. The button’s hover effect (button:hover) changes the color, signaling that it’s clickable.
Benefits of Hover Effects
- Improves visual feedback.
- Guides the user intuitively.
- Adds polish to the design.
Example Login Form in HTML Code
Here’s how the HTML and CSS come together for a fully functional login form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login Form</title>
<style>
/* Include the CSS code here */
</style>
</head>
<body>
<div class="login-container">
<h2>Login</h2>
<form>
<label for="username">Username</label>
<input type="text" id="username" name="username" required>
<label for="password">Password</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
</form>
</div>
</body>
</html>
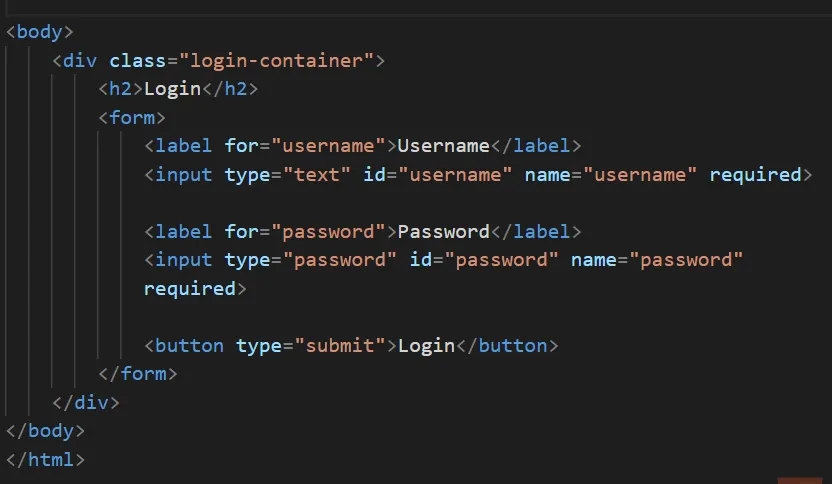
FAQs
How do I add more fields to the form?
You can add additional <label> and <input>tags for fields like email or phone number.
Can I customize the colors of the form?
Absolutely! Modify the CSS values for properties like background, color, and border.
How do I make the form mobile-friendly?
Use responsive design techniques, such as setting max-width and media queries in CSS.
How do I secure the Login Form in HTML Code?
Integrate server-side validation and SSL certificates to ensure data security.
Can I use this login form with JavaScript frameworks?
Yes, you can enhance functionality by integrating it with frameworks like React or Angular.