How to Pass Function as Props in React– In React, function props (also known as callback props) allow child components to communicate with their parent components. This technique is essential for handling events and updating the state dynamically. In this guide, we will explore How to Pass Function as Props in React with examples, explanations, and best practices.
What Are Function Props in React?
Function props in React are functions passed from a parent component to a child component as props. The child component can then call these functions to trigger specific actions or update the parent component’s state. Think of function props as a way for components to communicate and exchange data seamlessly.
Why Use Function Props?
Using function props is useful when:
- A child component needs to update the parent’s state.
- You want to handle events from the child component inside the parent.
- You need to maintain a single source of truth while keeping the UI flexible.
How to Pass a Function as Props in React
Let’s take a simple example where a parent component passes a function to a child component.
Parent Component (Passing Function as Props)
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const handleClick = () => {
alert('Button clicked!');
};
return (
<div>
<h1>Parent Component</h1>
<ChildComponent onClick={handleClick} />
</div>
);
};
export default ParentComponent;
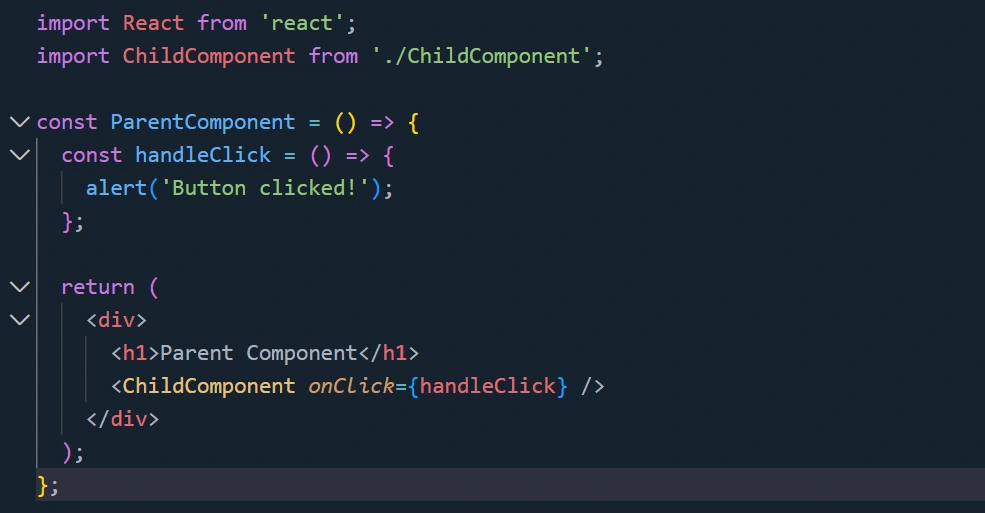
Child Component (Receiving and Using Function Props)
import React from 'react';
const ChildComponent = ({ onClick }) => {
return (
<div>
<h2>Child Component</h2>
<button onClick={onClick}>Click me</button>
</div>
);
};
export default ChildComponent;
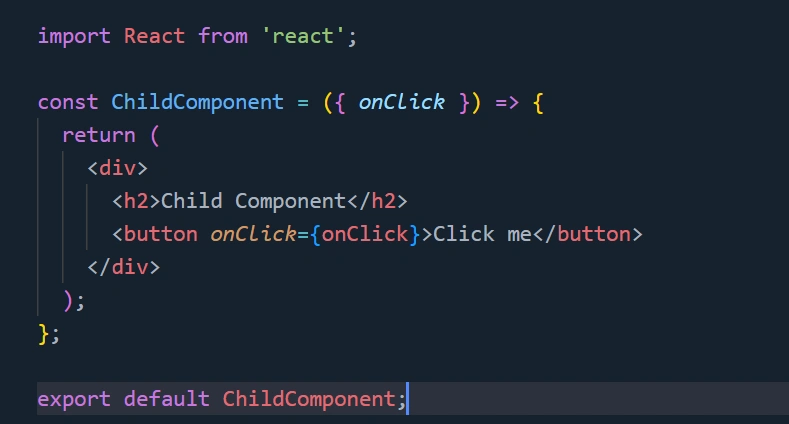
How It Works: How to Pass Function as Props in React
- ParentComponent defines the function
handleClick.
- It passes
handleClick
as a prop (onClick
) to the ChildComponent. - The ChildComponent uses
onClick
on the button. - When the button is clicked, it executes
handleClick
, triggering the alert.
Understanding the Parent-Child Communication
In React, data flows from parent to child using props. However, child components cannot directly modify parent data. Function props allow child components to notify parents when an action occurs.
Concept | Description |
---|---|
Function Props | Functions passed from a parent component to a child component as props. |
Parent Component | Defines and passes the function. |
Child Component | Receives and calls the function when needed. |
Callback Function | A function executed inside the child component but defined in the parent. |
Best Practices for Using Function Props
Here are some best practices when passing functions as props in React: How to Pass Function as Props in React
- Use Descriptive Function Names – Instead of onClick, use names like handleButtonClick.
- Keep Functions in the Parent Component – This maintains better state management.
- Use Arrow Functions – Prevent unnecessary re-renders when passing inline functions.
- Avoid Passing Anonymous Functions Directly – It can cause performance issues in large apps.
FAQs: How to Pass Function as Props in React
Can a child component pass data to a parent in React?
Yes! A child component can send data to the parent using function props. The parent defines a function that receives data and updates its state.
What happens if I pass a function as a prop without calling it?
If you pass a function as a prop without calling it, it remains available in the child component but does not execute until explicitly invoked.
Are function props the same as event handlers?
No, but they are often used together. Event handlers handle user interactions, while function props allow child components to communicate with the parent.
Can I pass multiple functions as props?
Yes! You can pass multiple functions as props and use them for different actions.
What is the difference between function props and state lifting?
Function props trigger actions in the parent, while state lifting moves shared state to the nearest common parent.