Props in React: Props in React, short for Properties, are used to pass data from one component to another. They allow components to be dynamic and reusable, enhancing the efficiency of React applications. Pro props enable communication between components, making it a fundamental concept in React development.
Understanding Props with an Example
To understand props, let’s take a simple example: passing a message
prop to a Greeting component
.
function Greeting(props) {
return <h1>{props.message}</h1>;
}
function App() {
return <Greeting message="Hello, React!" />;
}
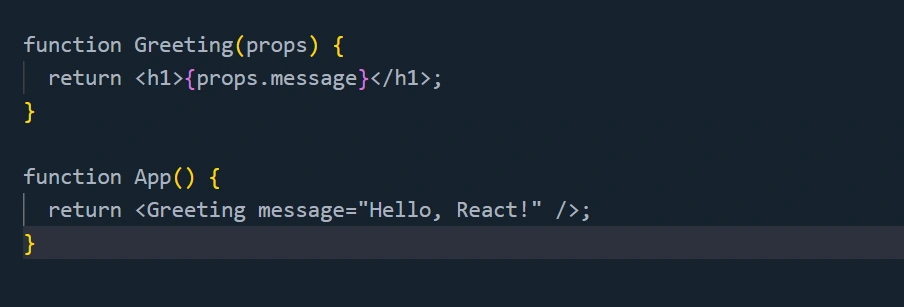
In the above code:
Greeting
component receivesmessage
as a prop.- The
App
component passes “Hello, React!” as a prop to Greeting. - The output will display: Hello, React!
How to Pass Props in React?
Props are passed like attributes in JSX syntax. The syntax follows:

For example:

Key Characteristics of Props in React
- Read-Only: Props are immutable, meaning they cannot be modified inside the component.
- Passed from Parent to Child: A parent component can send data to a child component using props.
- Enable Dynamic Content: Props allow the rendering of dynamic content.
Components Communication using Props
React follows a one-way data flow, meaning data moves from parent to child. Here’s an example demonstrating component communication:
function ChildComponent(props) {
return <h2>Received: {props.data}</h2>;
}
function ParentComponent() {
return <ChildComponent data="Hello from Parent!" />;
}
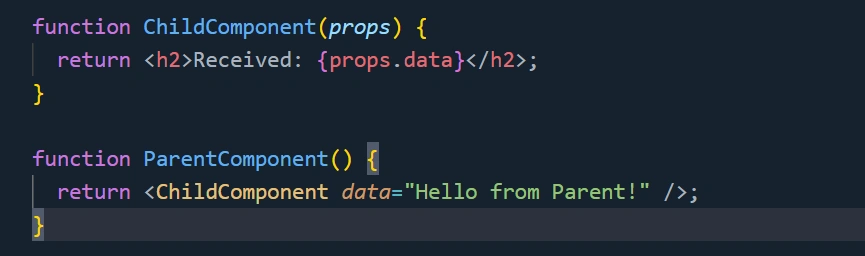
Props vs. State in React
Feature | Props | State |
---|---|---|
Mutability | Immutable | Mutable |
Ownership | Passed by parent | Managed within component |
Usage | Used for communication | Used for managing local data |
Common Use Cases of Props
- Passing data from a parent to a child component.
- Configuring components dynamically.
- Handling component reusability.
Frequently Asked Questions (FAQs)
Can we modify props inside a component?
No, props are immutable and cannot be modified inside the receiving component.
What is the difference between props and state?
Props are passed from parent to child, while the state is managed within a component.
How do props help in component reusability?
Props allow you to pass different values to a component, making it reusable in different scenarios.
Can we pass functions as props?
Yes, functions can be passed as props to handle events or trigger actions in child components.
Are props required in every component?
No, props are optional. Components without props can still function properly but may have static content.