Understanding call, apply, and bind in JavaScript- Mastering the concepts of call, apply,
and bind
in JavaScript is essential for any developer aiming to harness the true power of function invocation and object context. These methods provide flexibility in how functions are called, especially when dealing with this
references. Let’s explore these methods with examples, a comparison table, and frequently asked questions.
The call()
Method
The call()
method calls a function with a specified this
value and arguments provided individually.
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
const person = {
name: 'Alice'
};
greet.call(person, 'Hello', '!');
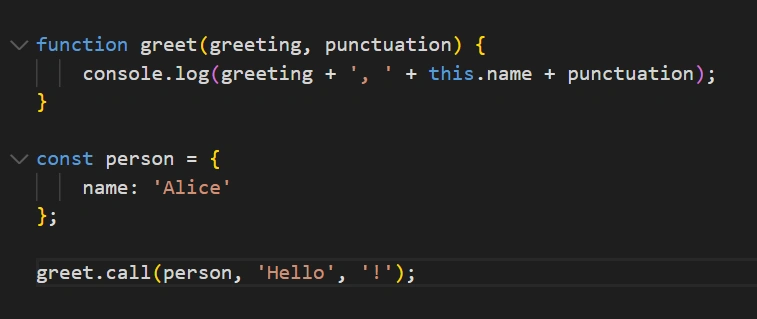
output

Key Points:
- Invocation: The function is invoked immediately.
- Arguments: Passed individually as separate parameters.
The apply()
Method
The apply()
method works similarly to call(),
but arguments are passed as an array instead of individually.
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
const person = {
name: 'Bob'
};
greet.apply(person, ['Hi', '?']);
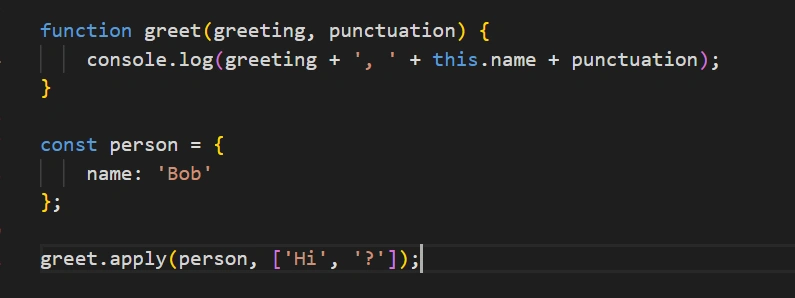
output

Key Points:
- Invocation: The function is invoked immediately.
- Arguments: Passed as a single array.
The bind()
Method
The bind()
method creates a new function with a specified this
value and optionally prepends arguments for later invocation.
function greet(greeting, punctuation) {
console.log(greeting + ', ' + this.name + punctuation);
}
const person = {
name: 'Charlie'
};
const greetCharlie = greet.bind(person, 'Hey');
greetCharlie('!!!');
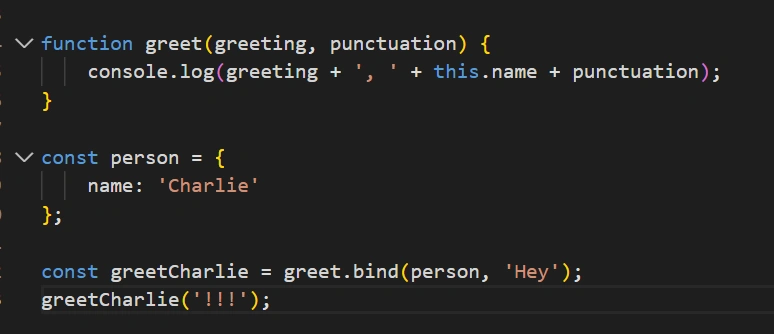
output

Key Points:
- Invocation: Returns a new function, allowing you to call it later.
- Arguments: Supports partial application (presetting some arguments).
Key Differences Between Understanding call, apply, and bind
Feature | call | apply | bind |
---|---|---|---|
Invocation | Invokes the function immediately. | Invokes the function immediately. | Returns a new function to call later. |
Arguments | Passed individually. | Passed as an array | Can partially apply arguments when binding. |
Use Case | Immediate invocation with context. | Immediate invocation with context. | Create reusable, context-bound functions. |
When to Use Which?
- Use
call:
When you need to invoke a function immediately and pass arguments individually. - Use
apply
: When you need to invoke a function immediately but already have arguments in an array. - Use
bind:
When you need a reusable function with a specificthis
context or want to apply arguments partially.
FAQ:
Q1. What happens if I don’t provide a context (this) in call, apply, or bind?
If this is not provided, it defaults to the global object (window in browsers, global in Node.js). In strict mode, it will be undefined.
Q2. Can I pass additional arguments with bind?
Yes! You can partially apply arguments when binding. These arguments will be prepended when the bound function is invoked.
Q3. Are there performance differences between these methods?
call
and apply
have similar performance as they invoke the function immediately. bind
, however, creates a new function, which might add slight overhead during creation but not during invocation.
Q4. Can I use apply if my function doesn’t accept multiple arguments?
Yes, you can still use apply, but you need to pass an empty array or one containing only a single argument.
Q5. Which method is better for handling variable-length arguments?
apply is ideal for variable-length arguments since it accepts an array, making it convenient to work with dynamic data.
Unlock the Power of call, apply, and bind
By mastering call, apply, and bind
in JavaScript, you can control function execution like a pro, manage this context effectively, and create reusable, context-aware functions. Try them out in your next project and watch your JavaScript skills soar!