Understanding Callback and Promise in JavaScript: JavaScript is a dynamic and versatile programming language. Two powerful features, callback functions, and promises, are integral to managing asynchronous operations effectively. Let’s explore these concepts using practical examples of Callback and Promise in JavaScript and simplify them into simple, actionable explanations.
Introduction to Asynchronous JavaScript
Asynchronous programming is like multitasking for JavaScript. It lets the code perform multiple operations simultaneously, avoiding blockages. Imagine cooking while waiting for water to boil—callbacks and promises are like setting timers to remind you to move to the next task.
What Are Callback Functions?
Definition of Callback Functions
A callback function is a function passed as an argument to another function. It gets executed after the operation is completed. Callbacks are one of the oldest ways to handle asynchronous tasks in JavaScript.
How Callbacks Work in JavaScript
Callbacks ensure that certain actions happen after an operation finishes. For example:
function fetchData(callback) {
console.log("Fetching data...");
setTimeout(() => {
callback("Data fetched!");
}, 2000);
}
fetchData((message) => console.log(message));
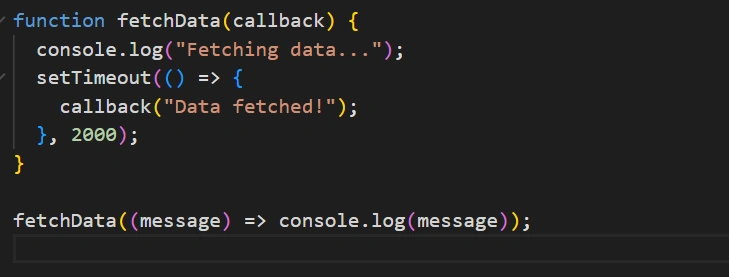
Output:

Examples of Callbacks in Action
Here’s another example using event listeners:
document.getElementById("btn").addEventListener("click", () => {
console.log("Button clicked!");
});

Understanding Promises in JavaScript
What Are Promises?
A promise is an object that represents the future completion or failure of an asynchronous operation. It’s like a pledge—it gets fulfilled, or something goes wrong.
States of a Promise
- Pending: Initial state; operation hasn’t completed yet.
- Fulfilled: Operation completed successfully.
- Rejected: Operation failed.
Here’s a promise in action:
const fetchData = new Promise((resolve, reject) => {
let success = true;
if (success) {
resolve("Data fetched successfully!");
} else {
reject("Error fetching data!");
}
});
fetchData
.then((message) => console.log(message))
.catch((error) => console.error(error));
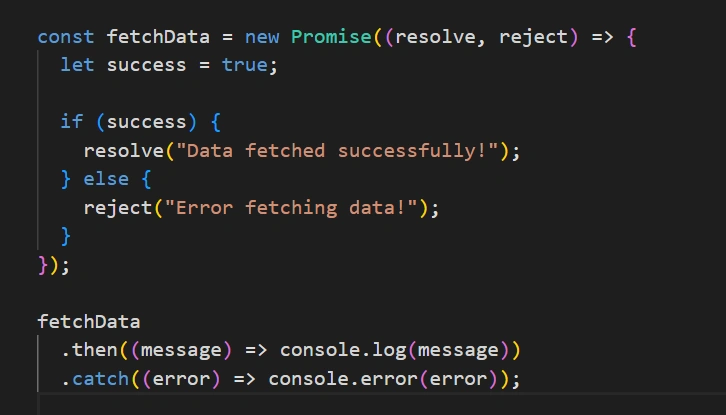
Promises vs. Callbacks: Key Differences
While callbacks work, they can sometimes lead to callback hell, a situation where nested callbacks make the code hard to read and maintain. Promises solve this problem by offering a cleaner syntax.
Code Example: Callback and Promise in JavaScript
const welcome = (name, callback) => {
console.log(`Hello, ${name}!`);
callback(name);
};
const goodbye = (name) => {
console.log(`Goodbye, ${name}!`);
};
welcome("Chris", goodbye);
// Output:
// Hello, Chris!
// Goodbye, Chris!
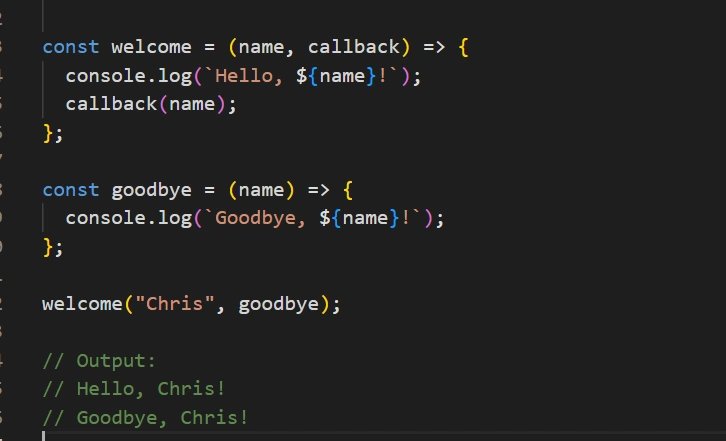
Step-by-Step Breakdown
welcome
Function: Accepts a name and a callback. It greets the user and triggers the callback.goodbye
Function: Logs a farewell message for the user.- Execution: The callback (
goodbye
) is executed after the welcome function completes.
Advantages of Using Promises
Promises make your code:
- Easier to read.
- Free from deeply nested structures.
- More efficient for error handling using
.catch().
Best Practices for Using Callbacks and Promises
Avoiding Callback Hell
Use modular functions and avoid excessive nesting. Break tasks into smaller chunks.
Using Async/Await for Simplicity
Async/Await is syntactic sugar over promises, making the code easier to read:
async function fetchData() {
try {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
Table: Callback and Promise in JavaScript
Feature | Callback Functions | Promises |
---|---|---|
Syntax | Nested and harder to read | Cleaner and easier to understand |
Error Handling | Must be handled in each callback | Centralized with .catch() |
Flexibility | Limited | More flexible and powerful |
Async/Await Support | Not supported | Fully supported |
Conclusion: Callback and Promise in JavaScript
Understanding callbacks and promises in JavaScript is crucial for handling asynchronous operations effectively. While callbacks provide the foundation, promises (and their async/await syntax) offer a more elegant solution for managing tasks in a cleaner, more maintainable way.
FAQs
What is a callback in JavaScript?
A callback is a function passed as an argument to another function and executed after the first function completes.
How do promises improve JavaScript code?
Promises provide a structured way to handle asynchronous tasks, improving readability and error handling.
What are the three states of a promise?
The three states are pending, fulfilled, and rejected.
Why is async/await better than promises?
Async/await makes code look synchronous, improving readability while maintaining asynchronous behavior.
Can I use callbacks and promises together?
Yes, but it’s better to avoid mixing them to keep the code clean and consistent.