What is an IIFE Function in JavaScript-IIFE (Immediately Invoked Function Expression) in JavaScript is a function that is defined and executed immediately after its creation. IIFEs are frequently used to avoid polluting the global scope and to create private variables that cannot be accessed from the outside. This pattern has become vital to JavaScript development, especially in scenarios requiring data encapsulation and memory efficiency.
Table of Contents
Understanding the Syntax of an IIFE
The syntax of an IIFE is straightforward. The function is wrapped in parentheses to turn it into an expression, and the parentheses at the end invoke the function immediately:
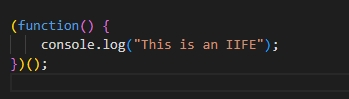
Here’s a breakdown of the syntax:
- Function Expression: The function is enclosed in parentheses to treat it as an expression.
- Immediately Invoked: The
()
at the end calls the function immediately. - Private Scope: The variables and functions defined inside the IIFE are inaccessible outside, providing a private scope.
Why Use IIFE Functions?
IIFE functions are particularly useful for:
- Preventing Global Scope Pollution: Variables and functions within an IIFE are not added to the global scope, reducing the risk of name collisions.
- Memory Efficiency: Since IIFE variables are local, they are destroyed after the function executes, freeing up memory.
- Encapsulation: IIFEs provide a way to create private data and methods, ensuring they cannot be accessed or modified from outside the function
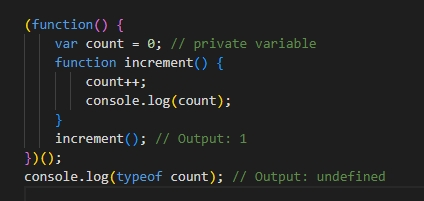
In this example, count
is not accessible outside of the IIFE, keeping it private.
IIFE Function with Parameters
You can also pass parameters to an IIFE to customize its execution:
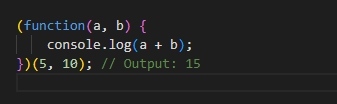
Passing arguments when invoking the function allows you to immediately perform operations without affecting the global state.
Using IIFE with Arrow Functions
ES6 introduced arrow functions, which can also be used to define IIFEs more concisely:
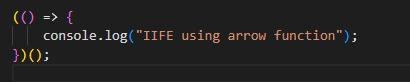
Arrow functions make the code even more compact and readable, while still providing the same benefits as a regular IIFE.
Practical Example: What is an IIFE Function in JavaScript
Let’s explore a practical scenario where an IIFE helps manage scope and functionality:
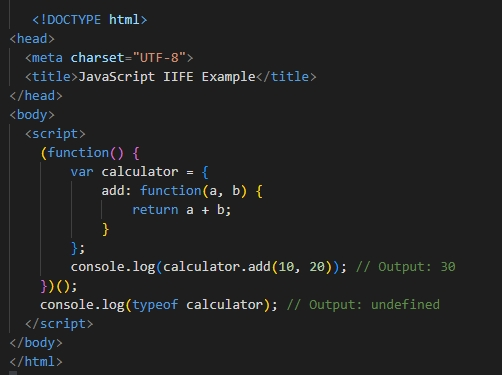
Explanation:
- Once the IIFE is completed, the calculator object is no longer accessible, as it’s confined to the IIFE’s scope.
- The calculator object is defined inside the IIFE, ensuring that it cannot be accessed globally.
- The function executes immediately, performing the calculation and outputting the result.
Benefits of Using IIFE Function in JavaScript
Benefit | Description |
---|---|
Encapsulation | Provides private scope, keeping variables and functions private. |
Global Scope Protection | Prevents polluting the global object with variables and functions. |
Memory Efficiency | Local variables are discarded after execution, freeing up memory. |
Anonymous Function Execution | Can execute code immediately, without needing to name or reuse the function. |
1. What is an IIFE in JavaScript?
An IIFE (Immediately Invoked Function Expression) is a function that is defined and executed immediately. It is useful for creating private scope and preventing global namespace pollution
2. Why should I use IIFE?
You should use IIFE to prevent global scope pollution, create private variables, and efficiently manage memory.
3. What is the difference between an IIFE and a Regular function?
Unlike regular functions, IIFE functions are invoked immediately after being defined, and they do not pollute the global namespace.