What is Hooks in React JS- React is a powerful JavaScript library used to build user interfaces efficiently. One of the most revolutionary features introduced in React 16.8 is Hooks. But what is Hooks in React JS? Simply put, Hooks allow you to use state and other React features without writing a class component.
In this article, we’ll explore the useState
Hook in React JS with an example, a breakdown of its functionality, and frequently asked questions.
Understanding Hooks in React JS
React Hooks are functions that let you “hook into” React state and lifecycle features from function components. The two most commonly used Hooks are:
useState
– Allows state management in function components.useEffect
– Manages side effects in function components.
These Hooks eliminate the need for class components while improving readability and maintainability.
The useState
Hook Explained
useState
is one of the most fundamental Hooks in React. It allows function components to have a local state. Instead of using this.state
in class components, function components can now use useState
to track state changes.
Basic Syntax of useState

state
– The current state value.setState
– Function to update the state.initialValue
– The starting value of the state.
Example: Counter App using useState
Let’s look at a simple counter application using useState
in React.
import React, { useState } from 'react';
function Example() {
// Declare a new state variable, "count"
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Example;
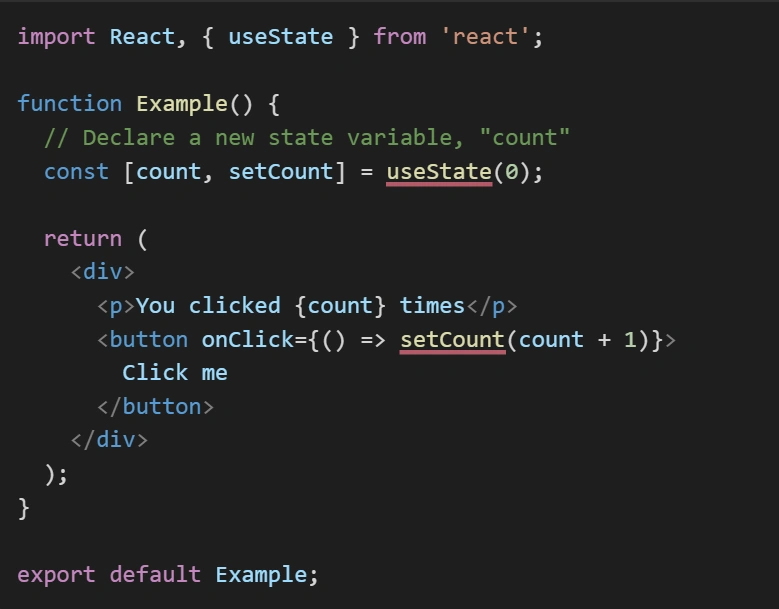
How It Works:
- We import useState from React.
- We declare a state variable count with an initial value of 0.
- The setCount function updates the state when the button is clicked.
- The UI updates dynamically to reflect the new state.
Advantages of Using Hooks in React JS
Feature | Benefits |
---|---|
No Class Components Needed | Simplifies code and improves readability |
Reusability | Can reuse Hooks across components |
Easier State Management | Manage component state without complexity |
Performance Optimization | Reduces component re-rendering |
Common Mistakes When Using useState
Not Updating State Correctly
Incorrect:

Correct:

Using the functional update form ensures the latest state is used.
Calling useState Inside Loops or Conditions
Hooks must be called at the top level of the function component to maintain consistency.
Conclusion
Hooks in React JS, particularly useState
, have revolutionized how developers manage state-in-function components. By using useState
, we can write cleaner, more concise, and reusable code without the complexity of class components.
FAQs
What is Hooks in React JS?
Explain details in the above article.
Why should I use useState
instead of class components?
useState
simplifies state management, improves code readability, and makes components easier to maintain.
Can I use multiple useState Hooks in a single component?
Yes! You can declare multiple useState Hooks to manage different states within the same component.
What happens if I call useState inside a loop or condition?
Calling useState inside loops or conditions can break the order of Hook calls and cause errors. Hooks should always be called at the top level of the function component.
Is useState synchronous or asynchronous?
useState updates are asynchronous. React batches multiple state updates to optimize performance.