What is Render in React JS- Rendering in React JS is a crucial process in class components that determines how UI elements appear on the screen. The render()
method is responsible for returning JSX, which React then converts into actual HTML and displays in the browser.
Understanding the Render Function in React JS
The render()
function is a part of class components in React. It is used to return JSX, which React then processes to update the UI efficiently. Unlike functional components that use hooks, class components rely on this method to define their structure and content.
Key Characteristics of the Render Method
- Returns JSX (JavaScript XML) to describe UI components.
- Reads
props
andstate
but cannot modify them. - Must be a pure function without side effects like HTTP requests.
- React calls
render()
automatically whenever there is a state or prop change.
How ReactDOM.render()
Works
ReactDOM.render()
is the function responsible for mounting React components into the actual DOM. It takes two arguments:
- The JSX or component to render (HTML code inside a React component).
- The target DOM element where the component should be displayed.
Example of ReactDOM.render()
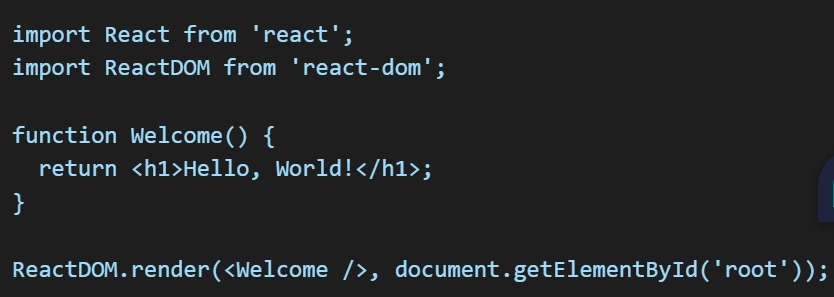
Here, the Welcome
component is rendered inside the HTML element with the id="root"
.
Rendering in Class Components
In class components, the render()
method must always return valid JSX or null. Here’s an example of What is Render in React JS:
import React, { Component } from 'react';
class Greeting extends Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default Greeting;
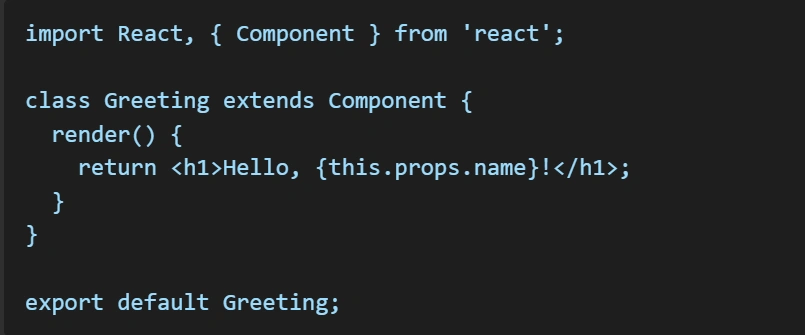
How it Works:
- The
render()
method readsprops
and returns JSX. - When
props.name
changes, React automatically re-renders the component.
Comparison of Functional and Class Component Rendering
Feature | Functional Component | Class Component |
---|---|---|
Uses render() method | No | Yes |
Uses Hooks | Yes (e.g., useState , useEffect ) | No |
State Management | Hooks | this.state |
Lifecycle Methods | No | Yes |
Common Mistakes to Avoid in Render() Method
Mutating State Directly:
this.state.count = 10; // Wrong
this.setState({ count: 10 }); // Correct
Using Side Effects in render():
fetch('https://api.example.com/data'); // Avoid in render()
Returning Multiple Elements Without a Wrapper:
return (
<h1>Hello</h1>
<p>Welcome to React</p>
); // Invalid JSX
Instead, use a fragment (<>…):
return (
<>
<h1>Hello</h1>
<p>Welcome to React</p>
</>
);
Conclusion: What is Render in React JS
The render() function in React JS plays a fundamental role in displaying UI elements in class components. It ensures the application reflects the latest state and prop changes. Understanding how ReactDOM.render()
and the render()
method work helps in building efficient and error-free React applications.
FAQs
What is the purpose of the render method in React?
The render() method is used to return JSX that describes the UI. It ensures the React component updates when there are changes in props or state.
Can we modify state inside render()?
No, modifying state inside render() is not allowed. Instead, use this.setState()
in lifecycle methods like componentDidMount().
How does React update the UI efficiently?
React uses a virtual DOM to compare changes and updates only the necessary parts of the actual DOM, making rendering more efficient.
Can we use multiple render methods in a class component?
No, a class component can have only one render() method, but it can return multiple JSX elements wrapped in a fragment.