What is State in ReactJS:- ReactJS is a popular JavaScript library for building user interfaces. One of its core concepts is state, which allows components to manage dynamic data and re-render accordingly. But what exactly is state in ReactJS, and how does it work? Let’s dive in!
Understanding State in ReactJS
State in ReactJS refers to an object that holds component-specific dynamic data. This data determines the behavior and rendering of the component. Unlike props, which are immutable and passed from a parent component, state is mutable and managed within the component itself.
Key Characteristics of State in ReactJS
- Encapsulated Data: State belongs to a specific component and cannot be accessed directly by others.
- Mutable: Unlike props, state values can be changed using the setState() method.
- Triggers Re-render: Any state update forces the component to re-render, updating the UI automatically.
- Asynchronous Updates: React batches multiple state updates for performance optimization.
Example of State in ReactJS
Here’s a basic example of how state is used in a React class component:
import React from 'react';
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
brand: 'Ford', // Example state property
};
}
render() {
return (
<div>
<h1>My Car</h1>
<p>Brand: {this.state.brand}</p>
</div>
);
}
}
export default MyComponent;
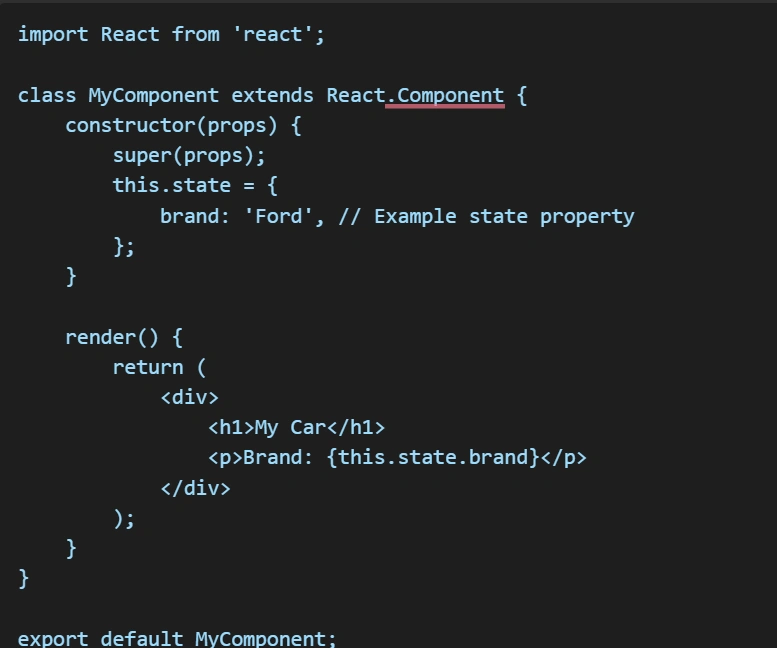
Breaking Down the Code
- Constructor Method: Initializes the component’s state inside
this.state.
- State Object: Holds the brand property.
- Using State in JSX: {
this.state.brand
} dynamically updates the UI. - Reactivity: If the state changes, React automatically updates the UI.
Managing State in React Components
Updating State with setState()
State should not be modified directly. Instead, use setState():

Handling Events to Update State
Example of updating state on a button click:
import React from 'react';
class Car extends React.Component {
constructor(props) {
super(props);
this.state = {
brand: 'Ford'
};
}
changeBrand = () => {
this.setState({ brand: 'Toyota' });
};
render() {
return (
<div>
<h1>My Car</h1>
<p>Brand: {this.state.brand}</p>
<button onClick={this.changeBrand}>Change Brand</button>
</div>
);
}
}
export default Car;
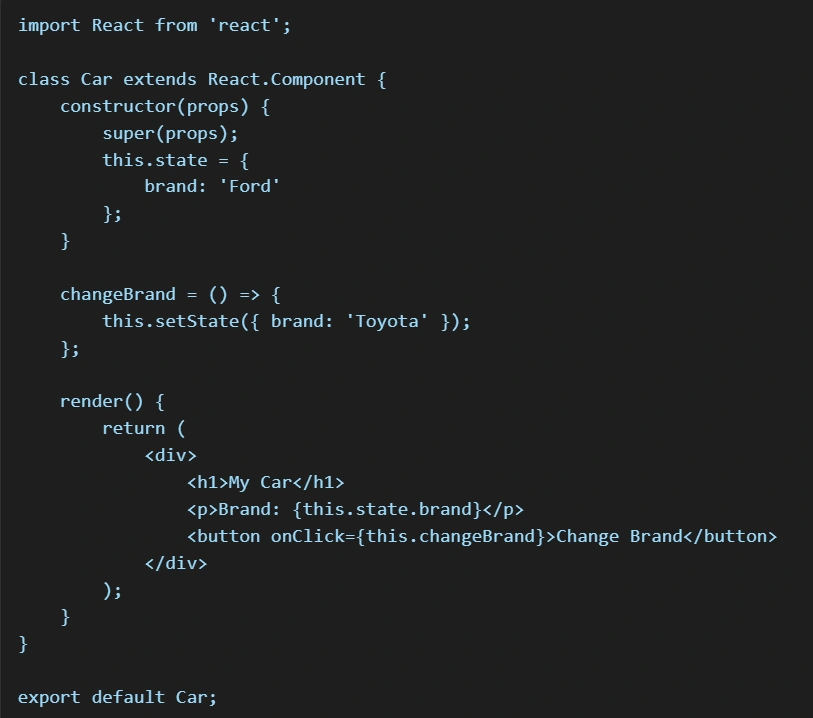
Using Functional Components and Hooks (useState)
Modern React encourages the use of functional components with the useState hook:
import React, { useState } from 'react';
function Car() {
const [brand, setBrand] = useState('Ford');
return (
<div>
<h1>My Car</h1>
<p>Brand: {brand}</p>
<button onClick={() => setBrand('Toyota')}>Change Brand</button>
</div>
);
}
export default Car;
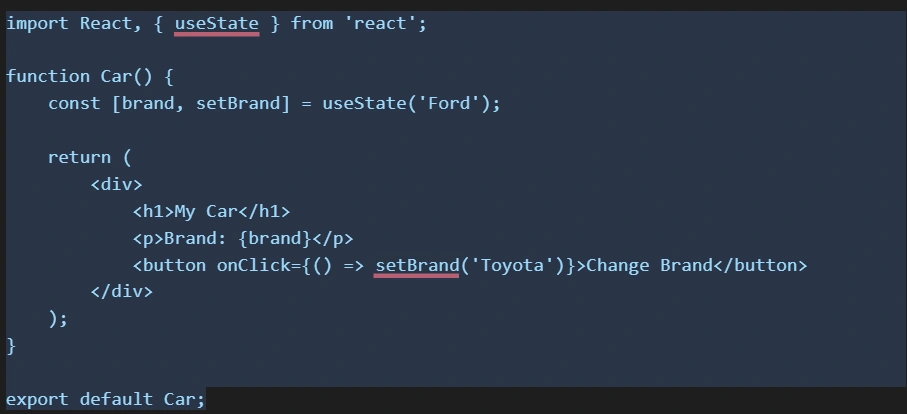
State vs Props: A Quick Comparison
Feature | State | Props |
---|---|---|
Mutability | Mutable | Immutable |
Ownership | Managed within the component | Passed from parent |
Updates | Causes re-render | Does not trigger re-render |
Usage | Used for dynamic changes | Used for passing data |
Best Practices for Using State in React
- Keep state minimal: Only store necessary data.
- Use functional updates: When the next state depends on the previous state.
- Avoid direct state mutations: Always use
setState()
. - Lift state up: If multiple components need access to the same state, move it to the nearest common ancestor.
Conclusion: What is State in ReactJS
State is a fundamental concept in ReactJS that allows components to handle dynamic data and reactivity. Understanding state management is crucial for building interactive applications, whether using class components with setState()
or functional components with useState
.
FAQs
What is state in ReactJS?
State is a JavaScript object that holds dynamic data and controls a component’s behavior in React.
How does state differ from props in React?
State is mutable and managed within a component, whereas props are immutable and passed from a parent component.
Why should I use setState()
instead of modifying state directly?
Directly modifying state won’t trigger a re-render, while setState()
ensures React updates the UI correctly.
Can I use state in functional components?
Yes, functional components use the useState
hook to manage the state.
What happens when state changes in React?
When the state changes, React automatically re-renders the component to reflect the updated data.