The Basics of this
in JavaScript
What is the bind() Method in JavaScript: The bind()
method in JavaScript is a powerful function designed to explicitly set the value of this for a function. This can be incredibly useful in situations where the context of this is lost, such as when a method is passed around as a callback or assigned to a different variable.
Context in JavaScript Functions
JavaScript functions run in a specific context. This context determines what this
refers to when the function executes
What is the this
Keyword?
The this
keyword represents the object that the function is currently operating on. Its value depends on how the function is called.
Global vs. Local Context
This refers to the window
object (or undefined in strict mode) in the global context. Depending on how the function is invoked, it can also refer to various objects inside a function.
Introducing the bind()
Method
What Does bind()
Do?
The bind()
method creates a new function where this
is explicitly set to the object passed as its argument. This ensures that the function always operates within the correct context.
Syntax of the bind() Method

- context: The object to bind this to.
- arg1, arg2, …: Optional arguments to pre-fill for the function.
When to Use bind()
- Callback Functions: Ensure a method retains the correct this when passed as a callback.
- Event Handlers: Bind event handlers to ensure they operate in the context of the component or object.
- Partial Application: Simplify function calls by pre-filling arguments.
By using bind(), you gain precise control over the behavior of functions and can avoid many of the common pitfalls caused by JavaScript’s dynamic this
behavior.
Examples of Using the bind() Method in JavaScript
const module = {
x: 42,
getX: function () {
return this.x;
},
};
const unboundGetX = module.getX;
console.log(unboundGetX()); // Output: undefined
const boundGetX = unboundGetX.bind(module);
console.log(boundGetX()); // Output: 42
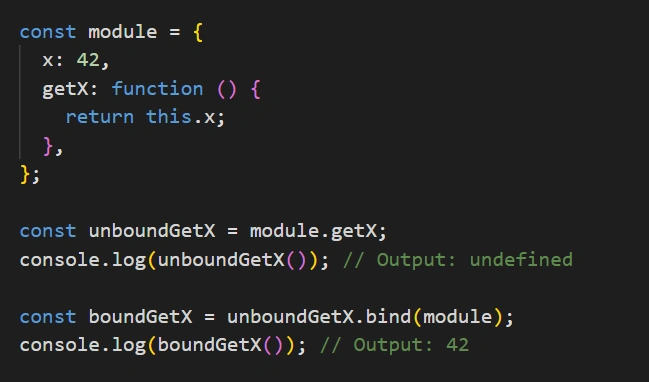
The bind()
method ensures that getX
uses module
as its context.
Example 2: Pre-filling Arguments with bind()
function multiply(a, b) {
return a * b;
}
const double = multiply.bind(null, 2);
console.log(double(5)); // Output: 10
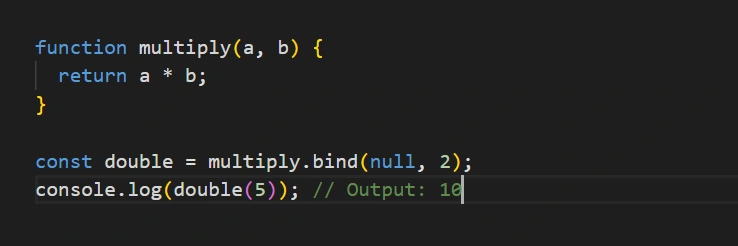
Here, bind()
creates a partially applied function that pre-fills the first argument as 2
.
Differences Between bind(),
call()
, and apply()
Feature | bind() | call() | apply() |
---|---|---|---|
Returns a new function | Yes | No | No |
Executes immediately | No | yes | yes |
Accepts arguments | Yes (partially or fully applied | Yes (individually) | Yes (as an array) |
Benefits and Limitations of bind()
Advantages
- Context Control: Ensures a function runs in the desired context.
- Partial Application: Simplifies function calls with pre-filled arguments.
Limitations
- Memory Usage: Creates a new function for every bind() call.
- Irreversibility: Once bound, the context cannot be changed.
Key Features of bind()
- Explicit Context Binding: You can tie a function to a specific object, avoiding unexpected this values.
- Returns a New Function: The original function remains untouched, while the new function has the specified context.
- Supports Partial Application: The bind() method allows pre-filling arguments to create specialized versions of functions.
Conclusion
The bind()
method in JavaScript is a powerful tool that solves context-related challenges, especially when working with this.
Whether you’re handling event listeners, creating partially applied functions, or ensuring consistent object methods, bind()
can significantly streamline your code.
FAQs
What is the main purpose of the bind() method in JavaScript?
The bind()
method ensures a function runs with the correct this
context, regardless of how it’s invoked.
Can I use bind() with arrow functions?
No, arrow functions do not have their own this and inherit it from their parent scope, so bind() has no effect.
What is partial application in JavaScript?
Partial application allows you to pre-fill some arguments of a function, creating a new function with fewer parameters.
How does bind() differ from call() and apply()?
bind()
creates a new function with bound context, while call()
and apply()
immediately execute the function with the specified context.
Are there any performance concerns with using bind()?
Excessive use of bind()
can increase memory usage because it creates a new function every time it’s called.